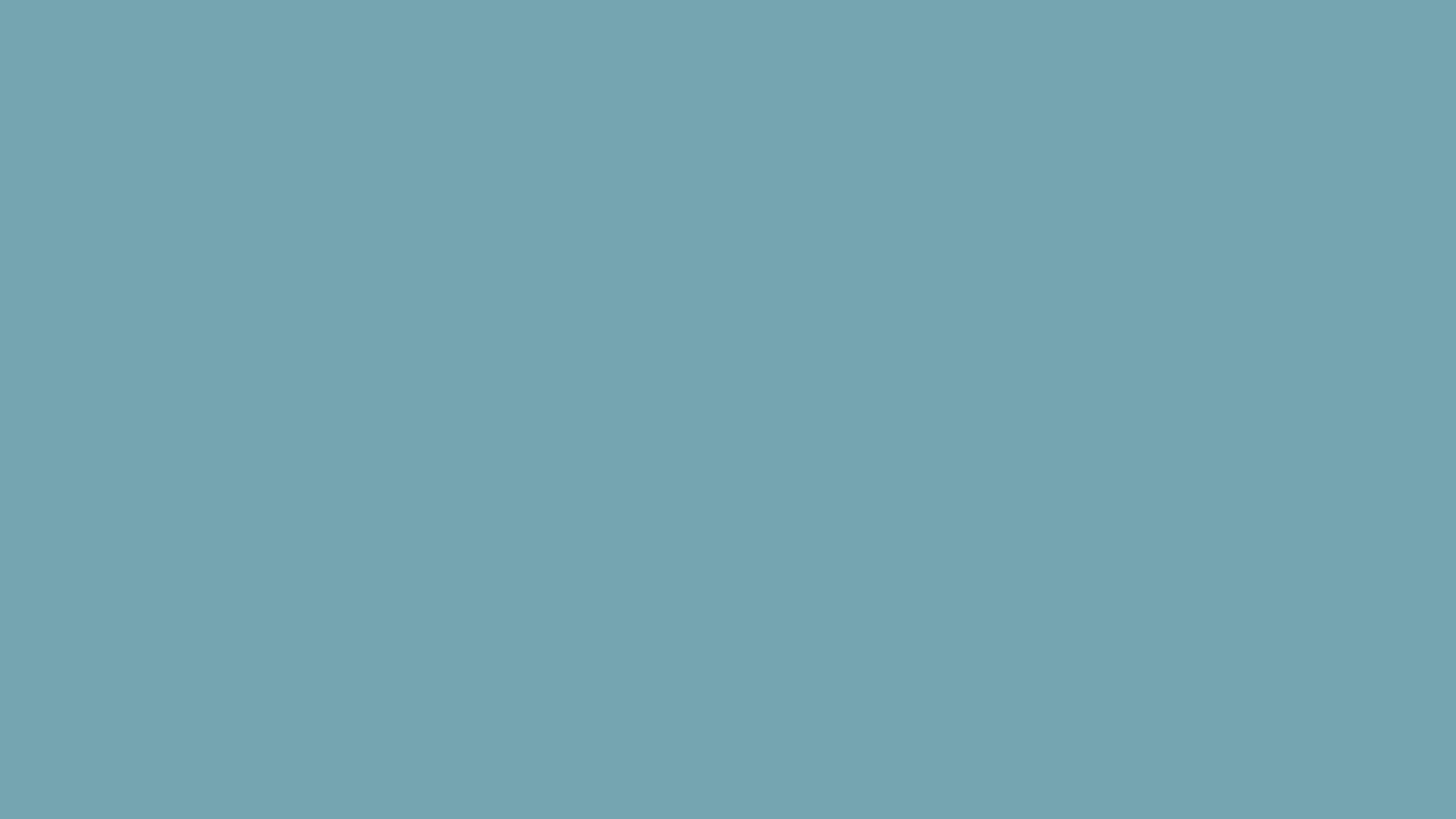
Converting days to years, months and days | JavaScript
A simple function for converting days to years, months and days.
JavaScript is what makes a website interactive. You can do so many cool things with vanilla JavaScript.
A simple function for converting days to years, months and days.
Commenting your code is important for readability and maintainability. Here's a couple of ways to add comments to your JavaScript code.
Sometimes you want to run a function a few seconds after the user has opened your website or similar. In this short guide, I'll show you how to do this.
There are a lot of different things you can do with JavaScript arrays. In this little guide, I'll go through some of them.
Sorting an array alphabetically or numerically is something we often do as programmers, but how do you do this using JavaScript?
Replacing strings in JavaScript can be done in many different ways. In this guide, I'll show you a couple of them.
In this little guide I'll show you how you can use JavaScript to check if a string starts with a certain word.
Splitting a string using JavaScript is something you do from time to time. But it's easy to forget the syntax, here's how you can split a string.
Let me show you how to use JavaScript to programmatically disable or enable a button.
A random string can be used for a lot of different purposes like password, filename, etc. But how do you generate one?
Generating random number using JavaScript isn't hard, but you need to know how to do it.
Sometimes you need to optimize your website by either using throttle or debounce, but what's the difference?
Every now and then, we want to check if an e-mail address is valid. Maybe you're building a user sign up or a newsletter registration form?
Sometimes you want to select an element inside another HTML element, but how do you do it?
JavaScript Reduce is a Higher-Order function and in this post I'll show you how to use it.
JavaScript Filter is a Higher-Order function and in this post I'll show you how to use it.
JavaScript Map is a Higher-Order function and in this post I'll show you how to use it.
You often want to check the value of an element or manipulate it some how. But if you do this without knowing if it exists or not, you might get an error.